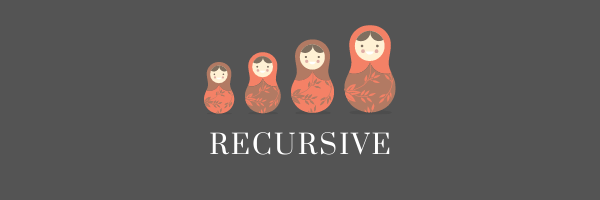
Lets Talk About Recursion / Recursive
21 April 2021
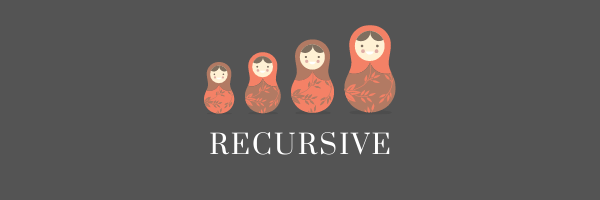
I always been amaze with recursive, what about you ?
A simple definition of recursive is
" Use function inside of function . "
Below is code for Countdown function using Recursive
function countdown(n){
// base case use this for break loop
if(n <= 0) {
return []
} else {
// call function again
let arr = countdown(n - 1)
arr.unshift(n)
return arr
}
}
countdown(10)
// [ 10, 9, 8, 7, 6, 5, 4, 3, 2, 1 ]
Below is code for display range of number using Recursive
function rangeOfNumbers(startNum, endNum) {
// base case use this for break loop
if( startNum === endNum ) {
return [startNum]
} else {
// call function again
const arr = rangeOfNumbers(startNum, endNum - 1)
arr.push(endNum)
return arr
}
}
rangeOfNumbers(1, 5)
// [ 1 , 2 , 3, 4, 5 ]